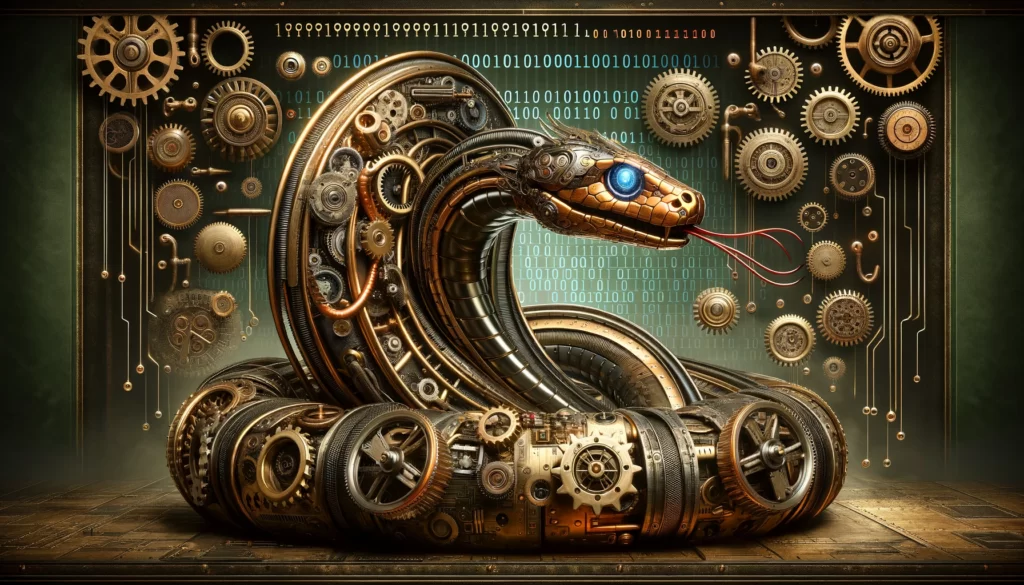
One of the most important things one can learn for hacking is getting comfy with programming. Python is a good language to start.
Another very important aspect of hacking is – SEARCH ENGINES. It stands to reason to combine searching and programming.
First, install the necessary library:
pip install googlesearch-python
This is how a request to google looks like:
try:
from googlesearch import search
except ImportError:
print("No module named 'google' found")
# to search
t=input()
query = t
for j in search(query, tld="co.in", num=10, stop=10, pause=2):
print(j)
Simple. Try it out.
Arguments of search function:
query: query string that we want to search for.
TLD: TLD stands for the top-level domain which means we want to search our results on google.com or google. in or some other domain.
lang: lang stands for language.
num: Number of results we want.
start: The first result to retrieve.
stop: The last result to retrieve. Use None to keep searching forever.
pause: Lapse to wait between HTTP requests. Lapse too short may cause Google to block your IP. Keeping significant lapses will make your program slow but itβs a safe and better option.
Return: Generator (iterator) that yields found URLs. If the stop parameter is None the iterator will loop forever.
How to search for python info:
If you don’t know what certain function does, get into a habit of searching it in proper locations!
One such location is: https://docs.python.org/3/
Another resource – good for checking additional modules documentations: https://pypi.org/project/
For example, in our program we use googlesearch module, and here is its description: https://pypi.org/project/google/, and from there we get to the homepage of the module author and its documentation: https://breakingcode.wordpress.com/2010/06/29/google-search-python/ and the source code: https://github.com/MarioVilas/googlesearch/blob/master/googlesearch/init.py
Yup, if you are going to program you will have to learn to navigate, search, effectively lookup for functions and modules, and sometimes even check their source codes (if no documentation is out there, but that happens rarely). Also, never forget to GOOGLE – GOOGLE stuff, you will find tons of tutorials with examples of specific functions.